1) First, you have to create mail account in Arvixe Control Panel (or your web hosting control panel)
Log in to Control Panel and select Mail -> Accounts and create new email account.
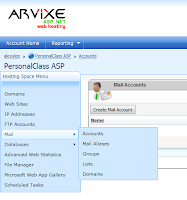
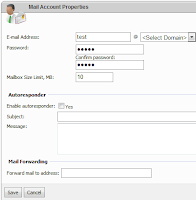
2) Once SMTP email account is created, you can send email in ASP.NET with the following code. Let's say your domain is TEST.COM; in that case your mail server will be mail.test.com in Arvixe.
MailMessage msg = new MailMessage(); msg.From = new MailAddress("admin@test.com"); msg.To.Add(new MailAddress("tom@gmail.com")); msg.Subject = "Meeting"; msg.Body ="Body message"; SmtpClient smtp = new SmtpClient("mail.test.com", 25); smtp.Credentials = new NetworkCredential("admin@test.com", "admin1234"); smtp.Send(msg);
In your ASP code-behind or any business class, you create MailMessage which includes From/To/Subject and Body message and send it to SMTP mail server through SMTP port 25.
You have to specify your mail server (mail.test.com) in SmtpClient constructor and since your SMTP server requires authentication, you also have to provide Credential. You can use your mail account information in credential username (admin@test.com in this example) and its password. If SMTP server requires credential but you don't specify username and password, the following exception will occur.
The SMTP server requires a secure connection or the client was not authenticated. The server response was: SMTP authentication is required.
3) The above code snippet will work but server name and user/password info are hardcoded. Not a good way. In ASP.NET, we can move those information to web.config as follows.
<system.net> <mailSettings> <smtp> <network host="mail.test.com" port="25" userName="admin@test.com" password="admin1234" /> </smtp> </mailSettings> </system.net>
And then C# code can be simplified like this.
private void SendSmtpMail(MailMessage message) { SmtpClient smtp = new SmtpClient(); smtp.Send(message); }
As you can see, you do not specify mail server name and port number. And credential information can come from web.config file.
4) Now, you can check your email. In our example, you are supposed to check your email from http://mail.test.com (in Arvixe case), assuming the domain is test.com.
nice article. if you are maintaining any template for email.. this may help you.
ReplyDeletehttp://aspnettutorialonline.blogspot.com/2012/05/email-template-from-flat-file-or.html
System.Net.Mail.SmtpException was caught
ReplyDeleteMessage=Failure sending mail.
Source=System
StackTrace:
at System.Net.Mail.SmtpClient.Send(MailMessage message)
at ********.UserControls.RecoverPassword.btnSend_Click(Object sender, EventArgs e) in **********************************************
InnerException: System.Net.WebException
Message=Unable to connect to the remote server
Source=System
StackTrace:
at System.Net.ServicePoint.GetConnection(PooledStream PooledStream, Object owner, Boolean async, IPAddress& address, Socket& abortSocket, Socket& abortSocket6, Int32 timeout)
at System.Net.PooledStream.Activate(Object owningObject, Boolean async, Int32 timeout, GeneralAsyncDelegate asyncCallback)
at System.Net.PooledStream.Activate(Object owningObject, GeneralAsyncDelegate asyncCallback)
at System.Net.ConnectionPool.GetConnection(Object owningObject, GeneralAsyncDelegate asyncCallback, Int32 creationTimeout)
at System.Net.Mail.SmtpConnection.GetConnection(String host, Int32 port)
at System.Net.Mail.SmtpTransport.GetConnection(String host, Int32 port)
at System.Net.Mail.SmtpClient.GetConnection()
at System.Net.Mail.SmtpClient.Send(MailMessage message)
InnerException: System.Net.Sockets.SocketException
Message=A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond ***.***.**.***:25
Source=System
ErrorCode=10060
NativeErrorCode=10060
StackTrace:
at System.Net.Sockets.Socket.DoConnect(EndPoint endPointSnapshot, SocketAddress socketAddress)
at System.Net.Sockets.Socket.InternalConnect(EndPoint remoteEP)
at System.Net.ServicePoint.ConnectSocketInternal(Boolean connectFailure, Socket s4, Socket s6, Socket& socket, IPAddress& address, ConnectSocketState state, IAsyncResult asyncResult, Int32 timeout, Exception& exception)
InnerException:
This Is My Error .. How Can I Solve It
Ga3far, this error is connection error. Somehow you cannot reach your SMTP server(***.***.**.***:25). The reason can vary. Please check to see smtp service is running and port 25 is open. You can try to connect with TELNET. Please also check your IP address is not local IP address (ie, use external DNS name).
ReplyDeleteHi,
ReplyDeleteThis works fine but in my case the receiver email id is a gmail account and the mails go to spam folder instead of inbox please help me in this
Hi Shama,
ReplyDeleteIt is gmail server that put your email into spam folder. Based on their criteria, gmail can consider your mail as spam. Please copy any of your Inbox mail content (valid, more complex content) to msg.Body and give it a try. This is not controlled by C# code.
Nice post very helpful
ReplyDeletedbakings
Wow nice post you made my problem solved in a minute :)
ReplyDeleteThanks from Aetherius http://aethtech.com/
Wow nice post you made my problem solved in a minute :)
ReplyDeleteThanks from Aetherius
Thanks a lot for providing valuable information. Nice blog, very helpful for all. Thanks for sharing best news with us. Well! if you want to get more information about Windows asp.net hosting then you can go to mywindowshosting.com
ReplyDeleteJust addition for you, this blog provide almost provide same tutorial about how to send email in asp.net. Hope this help.
ReplyDeleteHi.. Thanks for sharing.. I also have a reference on how to send email in asp.net . may be useful .
ReplyDeleteThis is nice post , thanks for sharing...!
ReplyDeleteweb hosting Bangalore
Arvixe Web Hosting Comparison Review - Choose the Best Web Hosting
ReplyDelete• Free ‘.com’ domain
• 99.9% uptime guarantee
• 24/7 technical support
• Unlimited email
• 60-day money-back guarantee
• WordPress integration
Great reasons to choose Arvixe
• Over 3 MILLION websites being hosted worldwide.
• 93% of users say they would recommend Arvixe.
Arvixe Web Hosting Discount
This blog is nice and very informative. I like this blog.
ReplyDeleteblog Please keep it up.
I like this web site very much, Its a really webcare360.com nice
ReplyDeletebillet to read and obtain info.