(2) New Project - WCF - [WCF Service Application]
(3) Delete IService1.cs and Service1.svc
(4) Add New Item - [WCF Service] item. Name it SimpleService.svc.
- - WCF client only can see interface (or class) with ServiceContract attribute.
- - WCF client only can use methods with OperationContract attribute. Properties, indexer, event cannot be used.
- - WCF can only use primitive data type and DataContract type.
- Regular CLR object reference cannot be used.
namespace SimpleWcfService { using System.Runtime.Serialization; using System.ServiceModel; [ServiceContract] public interface ISimpleService { [OperationContract] string SayHello(string name); [OperationContract] Customer UpdateCustomer(Customer customer); } [DataContract] public class Customer { [DataMember] public int ID { get; set; } [DataMember] public string Name { get; set; } } }
// SimpleService.svc.cs namespace SimpleWcfService { public class SimpleService : ISimpleService { public string SayHello(string name) { string v = "Hello " + name; return v; } public Customer UpdateCustomer(Customer customer) { customer.Name += "#"; return customer; } } }
<%@ ServiceHost Language="C#" Debug="true" Service="SimpleWcfService.SimpleService" CodeBehind="SimpleService.svc.cs" %>
<?xml version="1.0"?> <configuration> <system.web> <compilation debug="true" targetFramework="4.0" /> </system.web> <system.serviceModel> <behaviors> <serviceBehaviors> <behavior> <serviceMetadata httpGetEnabled="true"/> <serviceDebug includeExceptionDetailInFaults="false"/> </behavior> </serviceBehaviors> </behaviors> <serviceHostingEnvironment multipleSiteBindingsEnabled="true" /> </system.serviceModel> <system.webServer> <modules runAllManagedModulesForAllRequests="true"/> </system.webServer> </configuration>
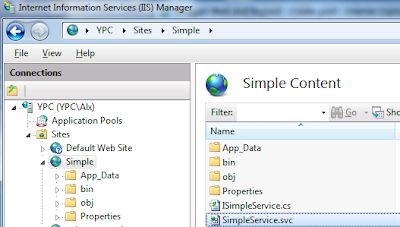
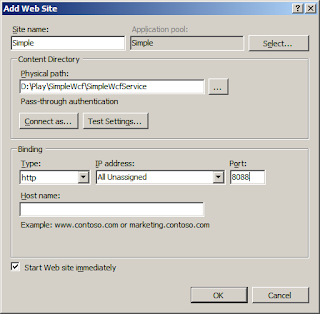
The following output will be shown when the WCF service is well hosted in IIS.
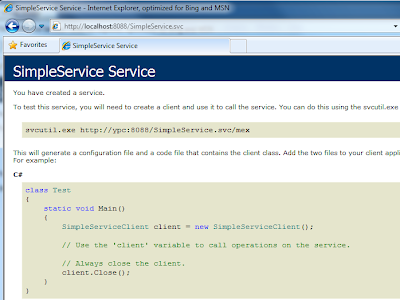
<system.serviceModel> <services> <service name="SimpleWcfService.SimpleService" behaviorConfiguration="behavior1"> </service> </services> <behaviors> <serviceBehaviors> <behavior name="behavior1"> <serviceMetadata httpGetEnabled="true"/> </behavior> </serviceBehaviors> </behaviors> </system.serviceModel>
In web browser, type http://localhost:8088/SimpleService.svc?wsdl
<system.serviceModel> <services> <service name="SimpleWcfService.SimpleService" behaviorConfiguration="behavior1"> <endpoint address="http://localhost:8088/SimpleService.svc" binding="basicHttpBinding" contract="SimpleWcfService.ISimpleService"></endpoint> <endpoint address="mex" binding="mexHttpBinding" contract="IMetadataExchange"></endpoint> </service> </services> <behaviors> <serviceBehaviors> <behavior name="behavior1"> <serviceMetadata /> </behavior> </serviceBehaviors> </behaviors> </system.serviceModel>
C:\Temp>svcutil.exe http://localhost:8088/SimpleService.svc/mex
Microsoft (R) Service Model Metadata Tool
[Microsoft (R) Windows (R) Communication Foundation, Version 4.0.30319.1]
Copyright (c) Microsoft Corporation. All rights reserved.
Attempting to download metadata from 'http://localhost:8088/SimpleService.svc/mex' using WS-Metadata Exchange or DISCO.
Generating files...
C:\Temp\SimpleService.cs
C:\Temp\output.config
Select File - [Add Service] and type WCF service URL. To call WCF service method, double a method from left pane and input any parameter and then click [Invoke] button. This makes a SOAP-based WCF service call and the response will be shown below pane.
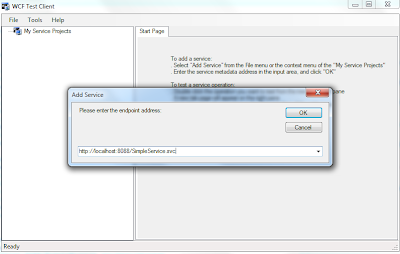
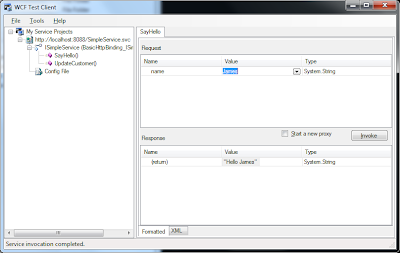
<system.diagnostics> <sources> <source name="System.ServiceModel" switchValue="All" propagateActivity="true"> <listeners> <add type="System.Diagnostics.DefaultTraceListener" name="Default"> <filter type="" /> </add> <add name="xml"> <filter type="" /> </add> </listeners> </source> <source name="System.ServiceModel.MessageLogging" switchValue="All"> <listeners> <add type="System.Diagnostics.DefaultTraceListener" name="Default"> <filter type="" /> </add> <add name="xml"> <filter type="" /> </add> </listeners> </source> <source name="XMLService.dll" switchValue="Warning, ActivityTracing"> <listeners> <add type="System.Diagnostics.DefaultTraceListener" name="Default"> <filter type="" /> </add> <add name="xml"> <filter type="" /> </add> </listeners> </source> </sources> <sharedListeners> <add initializeData="C:\Temp\mywcf.svclog" type="System.Diagnostics.XmlWriterTraceListener, System, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" name="xml" traceOutputOptions="LogicalOperationStack, DateTime, Timestamp, ProcessId, ThreadId, Callstack"> <filter type="" /> </add> </sharedListeners> <trace autoflush="true" /> </system.diagnostics>
No comments:
Post a Comment